useDebugValue
是一个 React 钩子,可让你将标签添加到 React 开发工具 中的自定义钩子。
¥useDebugValue
is a React Hook that lets you add a label to a custom Hook in React DevTools.
useDebugValue(value, format?)
参考
¥Reference
useDebugValue(value, format?)
在 自定义钩子 的顶层调用 useDebugValue
以显示可读的调试值:
¥Call useDebugValue
at the top level of your custom Hook to display a readable debug value:
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
参数
¥Parameters
-
value
:你想要在 React DevTools 中显示的值。它可以有任何类型。¥
value
: The value you want to display in React DevTools. It can have any type. -
可选
format
:一个格式化函数。当检查组件时,React DevTools 将调用格式化函数,并将value
作为参数,然后显示返回的格式化值(可以是任何类型)。如果不指定格式化函数,将显示原来的value
本身。¥optional
format
: A formatting function. When the component is inspected, React DevTools will call the formatting function with thevalue
as the argument, and then display the returned formatted value (which may have any type). If you don’t specify the formatting function, the originalvalue
itself will be displayed.
返回
¥Returns
useDebugValue
不返回任何东西。
¥useDebugValue
does not return anything.
用法
¥Usage
为自定义钩子添加标签
¥Adding a label to a custom Hook
在 自定义钩子 的顶层调用 useDebugValue
以显示 React 开发工具 的可读调试值
¥Call useDebugValue
at the top level of your custom Hook to display a readable debug value for React DevTools.
import { useDebugValue } from 'react';
function useOnlineStatus() {
// ...
useDebugValue(isOnline ? 'Online' : 'Offline');
// ...
}
当你检查它们时,这会为调用 useOnlineStatus
的组件提供一个类似于 OnlineStatus: "Online"
的标签:
¥This gives components calling useOnlineStatus
a label like OnlineStatus: "Online"
when you inspect them:
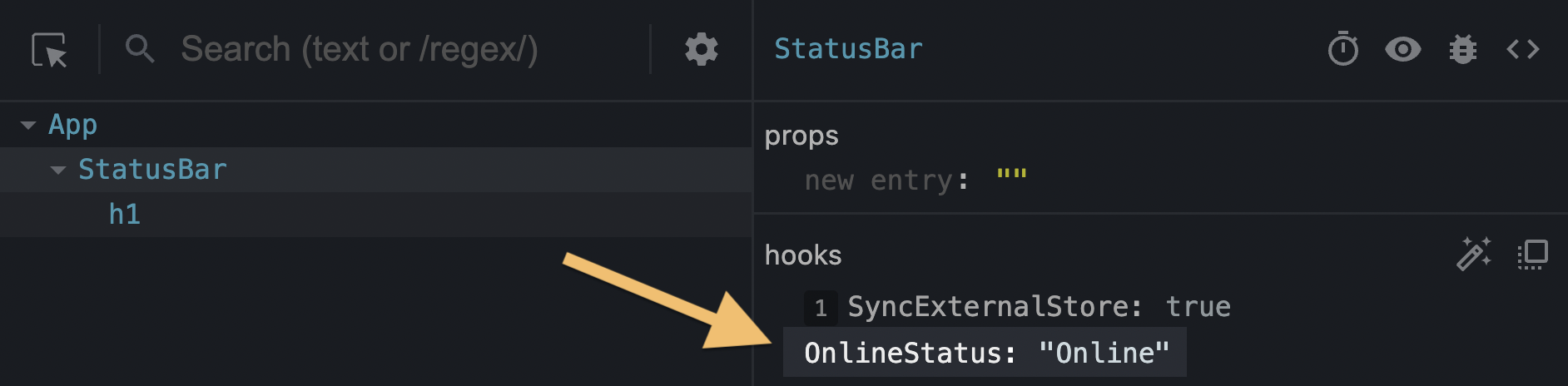
如果没有 useDebugValue
调用,则只会显示基础数据(在本例中为 true
)。
¥Without the useDebugValue
call, only the underlying data (in this example, true
) would be displayed.
import { useSyncExternalStore, useDebugValue } from 'react'; export function useOnlineStatus() { const isOnline = useSyncExternalStore(subscribe, () => navigator.onLine, () => true); useDebugValue(isOnline ? 'Online' : 'Offline'); return isOnline; } function subscribe(callback) { window.addEventListener('online', callback); window.addEventListener('offline', callback); return () => { window.removeEventListener('online', callback); window.removeEventListener('offline', callback); }; }
延迟调试值的格式化
¥Deferring formatting of a debug value
你还可以将格式化函数作为第二个参数传递给 useDebugValue
:
¥You can also pass a formatting function as the second argument to useDebugValue
:
useDebugValue(date, date => date.toDateString());
你的格式化函数将接收 调试值 作为参数,并且应该返回一个格式化显示值。当你的组件被检查时,React DevTools 将调用此函数并显示其结果。
¥Your formatting function will receive the debug value as a parameter and should return a formatted display value. When your component is inspected, React DevTools will call this function and display its result.
这使你可以避免运行可能代价高昂的格式化逻辑,除非实际检查了组件。例如,如果 date
是一个日期值,这将避免在每次渲染时对其调用 toDateString()
。
¥This lets you avoid running potentially expensive formatting logic unless the component is actually inspected. For example, if date
is a Date value, this avoids calling toDateString()
on it for every render.